Contents
C Programming Logic
Programming logic flow of program from one statement to another. They are used to regulate the execution order of the program statements. There are three types of program logic or control structure in programming. They are:
Sequential Structure
In this type of logic or structure, the program statements are executed one after another serially. Here, no condition or looping is used.
Flowchart of Sequential structure
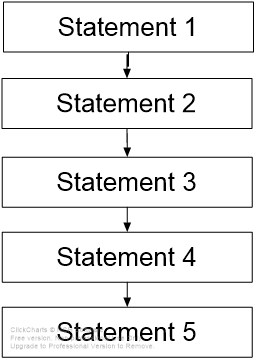
Example:
Program to calculate the area of a room
#include <stdio.h> #include <conio.h> void main() { clrscr(); int l, b, area; printf(“Enter length, Breadth”); scanf(“%d%d”, &l, &b); area = l * b; printf(“Area of Room = %d”, area); getch(); }
Formatted input/ output functions
The formatted input allows reading data from the keyboard and assigning them to a suitable variable. It also refers to input data that can be arranged in a particular format. Similarly, the formatted output allows writing or displaying data on the screen as output. It also refers to an output of data that can be arranged in a particular format.
a. scanf()
This is a formatted function that is used to input data in the C language.
Syntax:
scanf("control string, Specifier", arg1, arg2... ...);
Where control string refers to the field format in which data are to be entered.
Example:
scanf(“%d”, &age); scanf(“%f”, &amount); scanf(“%s”, &name);
b. printf() function
This is a library function that is used for formatted output in the C language.
Syntax:
printf("control string, specifier", arg1, arg2... ...);
where control string refers to the field format in which data are to be entered.
Example:
printf(“%d”, age); printf(“%f”, percent); printf(“%s”, name);
Unformatted Input/ Output functions
Unformatted functions do not allow the user to read or display data in the desired format. The unformatted library functions basically deal with a single character or string of characters.
- getchar(): This function reads a character from the standard input device i.e. keyboard.
Syntax: Char_var = getchar();
- putchar(): This function displays a character to the standard output device i.e. monitor.
Syntax: Putchar(char_var);
Some other functions of the C language
- clrscr(): This function is used to clear the screen in the C language. It can be used anywhere in the program but usually, it is used before the declaration level.
Syntax: clrscr(); example: clrscr();</pre></code> </li> <li><b>getch(): </b>This is an unformatted function that is used to pause a screen for a while immediately after executing the current program or action until the user press any character. It will wait till getting or pressing any character so that the result or output of the program will be seen clearly.<br> <pre><code class="c">Syntax: getch(); Example:getch();
Some important examples of Sequential logic programming techniques.
[Type the following program in C editing screen then compile/ execute and see the result]
a. Program to calculate and print simple interest according to given principal, rate, and time using C language.
#include <stdio.h> #include <conio.h> main() { float p, t, r, s; printf("Enter Principal "); scanf("%f", &p); printf("Enter Time "); scanf("%f", &t); printf("Enter Rate "); scanf("%f", &r); s = (p * t * r) / 100; printf("The Simple Interest of Princiapl: Rs. %0.1f, Time: %0.1f years, Rate: %0.1f is Rs %0.2f", p, t, r, s); getch(); }
b. Program to convert temperature centigrade into farenheight using C language.
#include <stdio.h> #include <conio.h> void main() { clrscr(); int c, f; printf("enter temperature in centigrade"); scanf("%d", &c); f = (9 / 5) * c + 32; printf("temperature in farenheight is %d", f); getch(); }
c. Program to check number palindrome using C language.
#include <stdio.h> #include <conio.h> int main() { int n, rem, result = 0, q; printf("Enter a number to check palindromen"); scanf("%d", &n); q = n; while (q != 0) { rem = q % 10; result = result * 10 + rem; q = q / 10; } if (result == n) { printf("It is a palindrome number"); } else { printf("It is not a palindrome number"); } return 0; }
d. Program to calculate the average of 3 numbers using C language.
#include <stdio.h> #include <conio.h> void main() { float a, b, c, d; printf("Enter first number "); scanf("%f", &a); printf("Enter second number "); scanf("%f", &b); printf("Enter third number "); scanf("%f", &c); d = (a * b * c) / 3; printf("The average of three numbers %0.1f, %0.1f, %0.1f is %0.2f", a, b, c, d); getch(); }
e. Program to reverse a string using C language.
#include <stdio.h> #include <conio.h> #include <string.h> main() { char ch[50]; printf("Enter a string to be reversedn"); scanf("%s", &ch); for (int i = strlen(ch) - 1; i >= 0; i--) { printf("%c", ch[i]); } getch(); }